In today’s digital age, adding a personal touch to your web applications can make a significant difference in user engagement. One way to achieve this is by creating custom message icons using JavaScript. In this blog post, we’ll walk you through a straightforward step-by-step procedure to infuse a dash of creativity into your messaging system.
Step1: Animated Message Icon
This code creates an interactive visual element within a container, featuring a dynamic honeycomb-shaped SVG animation alongside a message displayed using the <marquee>
tag, accompanied by a set of animated dots for added flair.
<div class="container"> <svg viewBox="0 0 374 374" fill="none" xmlns="http://www.w3.org/2000/svg"> <g clip-path="url(#clip0)"> <path d="M318.084 132.622C311.339 101.37 287.642 82 256.652 82C238.423 82 217.459 89.3248 197.954 102.509C191.574 106.904 182.642 106.904 176.079 102.672C155.298 88.9992 135.246 82 116.652 82C85.6626 82 61.9647 101.533 55.2199 133.111C44.2824 183.896 80.923 252.26 187.017 291C292.746 251.446 329.204 183.082 318.084 132.622Z" /> <path d="M187.466 0C187.366 0 187.166 0 186.866 0C85.666 0 3.36603 82.3 3.36603 183.5C3.36603 224.8 17.466 264.9 43.266 297.2L16.566 359.3C14.366 364.4 16.766 370.3 21.766 372.4C23.566 373.2 25.566 373.4 27.466 373.1L125.366 355.9C144.966 363 165.566 366.6 186.366 366.5C287.566 366.5 369.866 284.2 369.866 183C370.066 82.1 288.366 0.1 187.466 0ZM192.966 269.8C188.966 271.3 184.666 271.3 180.666 269.8C149.066 257.1 124.466 238.3 109.466 215.4C95.966 194.7 90.766 171.4 94.866 149.9C100.466 121.4 121.866 102.3 148.266 102.3C160.966 102.3 173.866 106.5 186.766 114.7C199.366 106.5 212.466 102.3 225.066 102.3C251.866 102.3 272.866 120.9 278.466 149.6C286.066 188.8 262.666 241.3 192.966 269.8Z" /> </g> <defs> <clipPath id="clip0"> <rect width="373.232" height="373.232" fill="white" /> </clipPath> </defs> </svg> <div class="dots"> <div class="dot"></div> <div class="dot"></div> <div class="dot"></div> </div> <div class="message"> <marquee> Honey we need to talk... </marquee> </div> </div>
Step2: Notification Interface with Dynamic Elements
This CSS code defines a visually pleasing notification container with a honeycomb-shaped SVG, animated dots, and a scrolling message. The design showcases a clean layout, vibrant colors, and subtle shadows for a polished user interface.
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600;700&display=swap"); *, *::after, *::before { margin: 0; padding: 0; -webkit-box-sizing: inherit; box-sizing: inherit; } body { -webkit-box-sizing: border-box; box-sizing: border-box; font-family: "Poppins", sans-serif; line-height: 1.7; position: relative; height: 100vh; background-color: #6d8e69e5; } .container { position: absolute; left: 50%; top: 50%; -webkit-transform: translate(-50%, -50%); transform: translate(-50%, -50%); width: 150px; height: 150px; border-radius: 40px; background-color: #ffffff; display: flex; transform-origin: left; box-shadow: 0px 5px 15px 2px #27cccc; svg { height: 90px; width: 90px; position: absolute; left: 50%; top: 50%; transform: translate(-50%, -50%); fill: #1e88e5; } .dots { display: flex; position: absolute; left: 50%; top: 52%; transform: translate(-50%, -50%); .dot { width: 10px; height: 10px; background-color: rgb(255, 255, 255); border-radius: 1000px; margin-right: 6px; &:last-child { margin-right: 0; } } } .message { width: 120px; height: 36px; background-color: #27cccc; overflow: hidden; position: absolute; right: -10px; top: -10px; border-radius: 50px; display: flex; padding: 5px 0; flex-wrap: nowrap; box-shadow: 0px 5px 15px 2px #d32f2f62; marquee { color: white; } } } .credits { position: absolute; right: 0; bottom: 0; padding: 20px; color: #423d36; font-weight: 500; p { a { font-weight: 600; text-decoration: none; color: #1f1d1a; } } }
Step3: Dot Animation with GSAP in JavaScript
This JavaScript code utilizes GSAP (GreenSock Animation Platform) to create an engaging animation for each dot element. The dots gracefully move up and down with a staggered effect, enhancing the visual appeal of the user interface.
// Get all the dots from the document let dots = document.querySelectorAll(".dot"); // We will be adding a delay of 0.2 between animation associated with each dot let delay = 0.2; // Function that will animate our dots. It takes the element and the delay. const animate = (el, delay) => { gsap.to( el, { ease: Linear.ease, translateY: -10, // Move the dot -30px on Y axis yoyo: true, // If true, playback will alternate forwards and backwards on each repeat repeat: -1, // how many times the animation should repeat (-1 is infinite) duration: 0.5, // the speed of the animation delay: delay, // time after which the animation starts }, 0 ); }; // Iterate over each dot and run the animate method, and also increment the delay by '.2s'. dots.forEach((dot) => { animate(dot, delay); delay += 0.2; });
Message Icons: Adding Icons to Messages with JavaScript Demo
Creating custom message icons using JavaScript is a simple yet effective way to add a personal touch to your web applications. By following these step-by-step instructions, you can enhance user experience and make your messaging system stand out.
You Might Be Interested In:
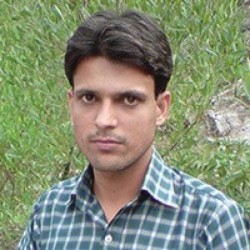
Ashfaq Ahmed
Ashfaq Ahmed is a freelance WordPress developer and owner of codeconvey.com. I developed modern and highly interactive websites. I always focus on improving my development and design skills.